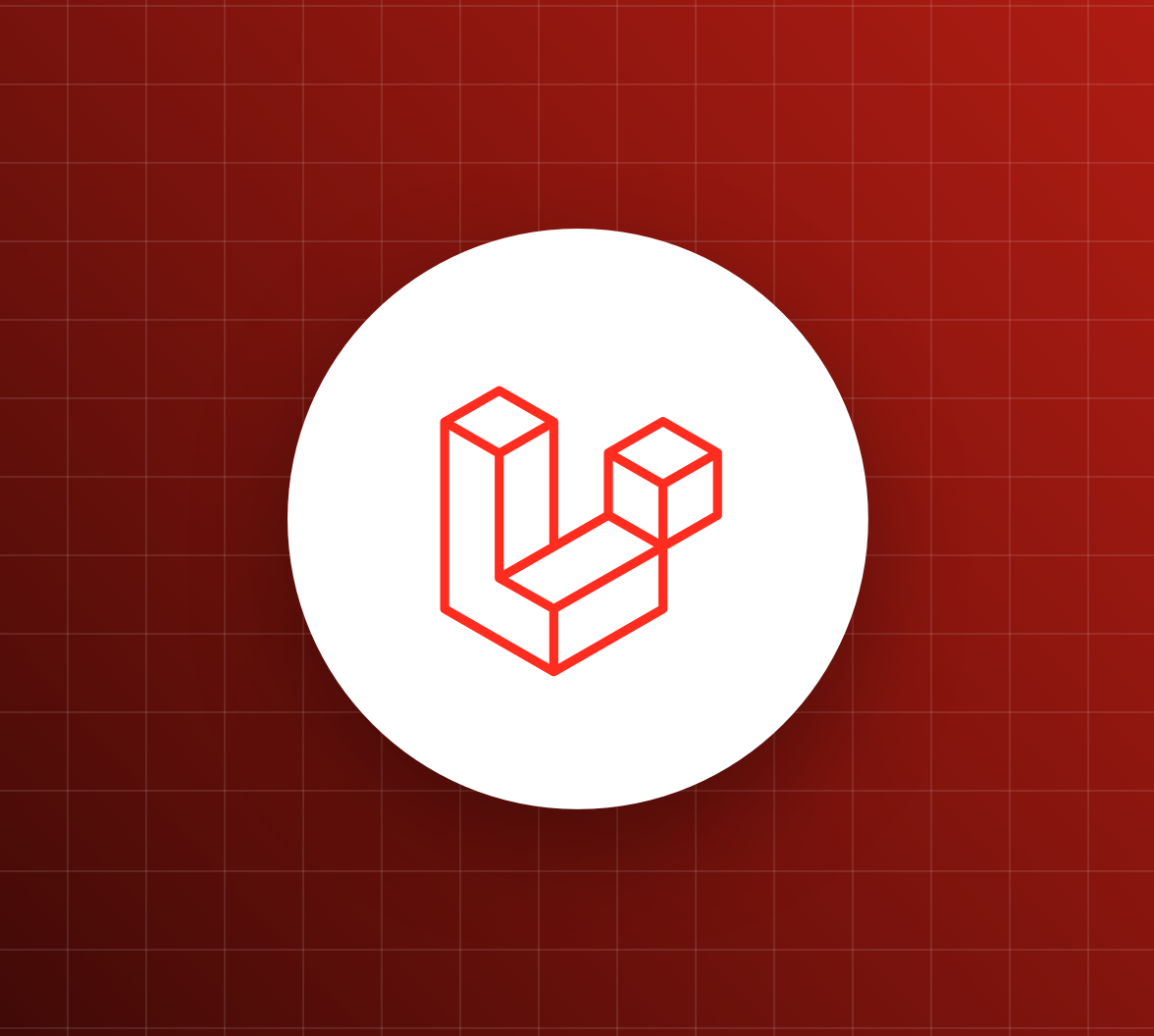
Contents |
---|
Do you find your code runs well the first, second, third time, but fails in production? Sometimes the unique but chances are assumed the data would be of “x” kind and actually it was “yyyxz” kind.
Null check
We’ve all made this mistake. Never assume a value won’t be null!
function getPikachusTrainer() {
$pokemon = Pokemon::where(“name”, “Pikachu”)->first();
return $pokemon->trainer->name;
}
Will return an error:
Trying to get property of non-object
But a simple check:
If ($pokemon) {
$trainer = Trainer::where(“name”, $pokemon->name);
} else {
return “sorry, no trainer found”;
}
Will allow us to avoid the issue.
Empty Check
The above works because it is an object and laravel returns null if a query can’t find anything. For arrays, we should not assume the array contains more than 0 elements.
foreach ($bagOfPokeballs as $key => $pokeball) {
print($key . “contains the following Pokemon” . $pokeball->name);
}
The above code will cause a similar error: “Trying to get property “name” of non-object”
In that case we can just add !empty($array) to our null check:
If (!empty($bagOfPokeballs) && $bagOfPokeballs) {
foreach ($bagOfPokeballs as $key => $pokeball) {
print($key . “contains the following Pokemon” )
print($pokeball->name)
}
}
Zero Check
Just like arrays, we should not assume integers are > 0. Especially since Laravel/PHP will return an error when divided by zero.
Divide By Zero Error
Thankfully, the way to avoid this is easy as pi!
if ($numBattles && $numBattles > 0) {
$percentageVictories = (($numWins / $numBattles) * 100)
}